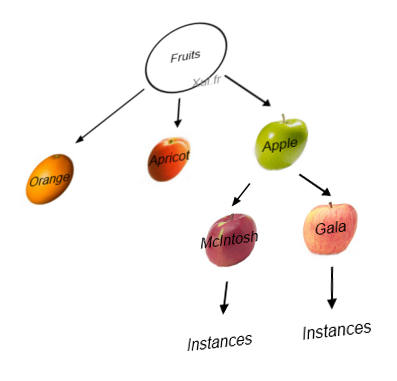
Dynamic objects in JavaScript
JavaScript has modernized object-oriented languages with dynamic construction. There is no such classes as in the C language, where methods and attributes are first defined and then used as models to create instances.
A JavaScript object is syntactically defined as a function, which is itself a first instance and that is cloned to create more instances.
In addition, this structure is dynamic, methods (in fact inner functions) and attributes may be added during the execution of the script.
The main question is whether a method or attribute is dynamically added to an object, they become available for derived objects, what we will see.
An object is defined as a function
The object is created by defining a constructor and by assigning it to an identifier. The this operator defines a variable as attribute and a function as method.
function fruit(price)
{
var color = "red";
this.price = price;
}
The constructor
may have arguments, and it contains properties.
It is assigned with the new keyword:
myfruit = new fruit(120);
It is possible to create an object by assigning a literal consisting in list of properties/values separated by a comma:
myfruit = { price:120, color:"red" };
Demo of static assignment:
myfruit = {
price:120,
color:"red",
orange: {
price:25
}
};
document.write("assignement of a literal object: ");
document.write(myfruit.orange.price);
Instance is created with the new operator
function fruit(v)
{
var color = "red"
this.price = v;
}
var myfruit=new fruit(120)
document.write(myfruit.price);
The color attribute can not be called because it is declared as a local variable. It is a private attribute (discussed below)
The instance can be declared directly with the definition of the object providing a value is assigned to the parameters if you want to access it after. Example:
var myfruit = new fruit(p = 200)
{
this.price = p;
}
document.write(myfruit.price);
Properties of an object may be added during the processing
An object can be defined as a list of properties, equivalent to the attributes of a class.
You can add a property in accordance with the following syntax:
objectname.propertyname = xxxx
The property is accessed according to the same syntax. Example for the object fruit which has a price property:
alert(fruit.price);
An object may be redefined dynamically with the prototype property
You can add a property directly to an already defined object. To dynamically add a property and that it is used by all clones derived from the same object, even if they are created before the property is added, use the reserved word prototype.
fruit.prototype.origin = "Europa";
The fuel property belongs to myfruit and all other instances defined from fruit.
Example of inheritance in JavaScript and retroactive modification of the prototype (and all its instances):
var myfruit = new fruit(p = 200)
{
this.price = p;
}
apple = myfruit;
document.write(apple.price);
fruit.prototype.origin="Europa";
document.write("origin=" + truck.origin);
An object can contain other objects assigned as values of attributes
A property can
be another object. In
this case we access properties of the inner object by a chain of identifiers
separated by a dot.
For example, if
the object myfruit contains an object x which has the constructor
orange with the property price, one can reach price
by:
function orange(p)
{
this.price = p;
}
x = new orange(25);
myfruit.orange = x;
document.write("price orange: ");
document.write(myfruit.orange.price);
An object can be incorporated into an another object as a literal:
myfruit = {
this.price:120,
this.color:"red",
this.orange : {
this.price:25
}
};
The object myfruit contains the object so defined too with a literal. Take note of the comma separating the items instead of semi-colons.
Values of attributes are accessed as in an array
Values of properties are accessed by name, as above or through their order in the list of properties of the object, as with the elements of an array, provided they the object is part of a HTML document.
If the object is not part of the HTML document, you can access the value by the name of the properties, or by an index if the value has been added at a position.
For example, if one assigns a value by a position:
fruit[4] = "demo";
We access the value in the same way:
alert(fruit[4]);
An object corresponds to an ambivalent array, with ordinal elements or properties, unlike PHP which uses numbers both as keys and ordinals, which makes the contents of array sometimes indeterminable.
Demo of accessing throught index:
fruit[1] = "demo";
document.write("value: ");
document.write(fruit[1]);
Methods are added to an object statically or dynamically
We can declare a function in another function and this is equivalent in JavaScript to define a method in an object.
To use a method, we must declare an instance of the object, it can not be used directly. Note that it is possible to make a static object, as discussed below.
Example:
function outer()
{
x = 10;
this.inner = function (y)
{
return y * 2 + x;
}
}
var o = new outer();
document.write(o.inner(50));
And we can add an attribute or a method dynamically:
outer.x2 = 10;
outer.inner2 = function(y)
{
return y * 2 + x + this.x2;
}
document.write(outer.inner2(50));
If we declare the method dynamically, attributes added dynamically must be referenced with the keyword this.
You may also declare attributes and methods statically inside an associative array:
var a = {
x : 10,
inner : function(y)
{
return y * 2 + this.x;
}
}
document.write(a.inner(50));
So they may be referenced directly with the static object. You can not create an instance of array, but you can assign it to variables, the effect is the same.
Methods and attributes are private or public
To create private members, we limit the scope to the object, so we use a variable with a var declaration and even for a function we assign it to a local variable.
function apple()
{
var privattribute = 0;
var privfun = function(x)
{
alert(x);
}
}
Instead, to create members that can be called outside the object, we gives them a global scope and the this prefix.
function apple()
{
this.color = "Red";
this.destination = function(y)
{
return (y + " " + this.color);
}
}
Example of using these public attributes and methods ...
var obj1 = new apple();
obj1. = "green";
var result = obj1.destination("Hello");
document.write(result);
More examples are given in the chapters about builtin objets (return to the summary).
See also:
- This and new, a close relationship, how a function becomes object.
- How to copy a JavaScript object.