Dynamic images with Image Map
In the part one, we saw how to display information about objects in an image and how when you click on the elements of an image that can show another page or another image.
We will develop this last point, and when you click on an object in the picture, the picture is replaced by an image of this object only.
Two methods may be employed: either using a bookmarklet, or by adding an onClick event handler.
The code of the image remains the same in both cases:
<img id="img1" src="firstphoto.jpg" width="500" height="540" border="0" usemap="#map1" >
And the basic image map code is the same too:
<map name="map1">
<area href="secondphoto.jpg" shape="circle" coords="240,180,140">
<area href="collar.jpg'" shape="rect" coords="60,330,440,520" >
</map>
You can access the image tag, by its ID:
document.getElementById("img1").src="new url";
or with the DOM:
document.images[0].src="new url";
Adding a onClick event
The event is added to the area tag, and we assign an action that changes the source of the img tag, to replace the image.
We also have to neutralizes the href attribute with a void bookmarklet: javascript: void (0).
<map name="map1">
<area href="javascript:void(0)" shape="circle" coords="240,180,140"
onClick="document.images[0].src = 'https://www.xul.fr/images/secondphoto.jpg'">
<area href="javascript:void(0)" shape="rect" coords="60,330,440,520"
onClick="document.images[0].src = 'https://www.xul.fr/images/collar.jpg'">
</map>
Using a bookmarklet
In this case the URL is replaced by a JavaScript function (see the article bookmarklet).
The bookmarklet code is as follows:
javascript:(
function()
{
document.getElementById('img1').src='https://www.xul.fr/images/asin1.jpg';
}
)()
It will be compacted in the href attribute:
<map name="map1">
<area href="javascript:(function(){document.getElementById('img1').src=
'https://www.xul.fr/images/secondphoto.jpg';})()" shape="circle" coords="240,180,140">
<area href="javascript:(function(){document.getElementById('img1').src=
'https://www.xul.fr/images/collar.jpg';})()" shape="rect" coords="60,330,440,520">
</map>
Limitations
The replacement is irreversible because the image map remains the same:
- We can not use the back button.
- The same areas are clickable on the new image.
To go further, we must dynamically assign a new image map to each new image displayed.
Example of dynamic Images
A picture may be replaced by another when you click on an object, a person, in the original image.
In this demonstration, the image map is not replaced for the new image appears.
The code uses two different methods to replace the image with ID and with the DOM. This has nothing to do with the images, it is just to demonstrate the two processes.
The code:
<map name="map1">
<area href="javascript:(function(){
document.getElementById('img1').src='https://www.xul.fr/images/asin1.jpg';})()"
shape="circle" coords="240,180,140">
<area href="javascript:void(0)"
shape="rect" coords="60,330,440,520"
onClick="document.getElementById('img1').src = 'https://www.xul.fr/images/collar.jpg'">
</map>
Click either on the face to replace the photo of the actress or the collar to display instead of the photo, an image of a necklace.
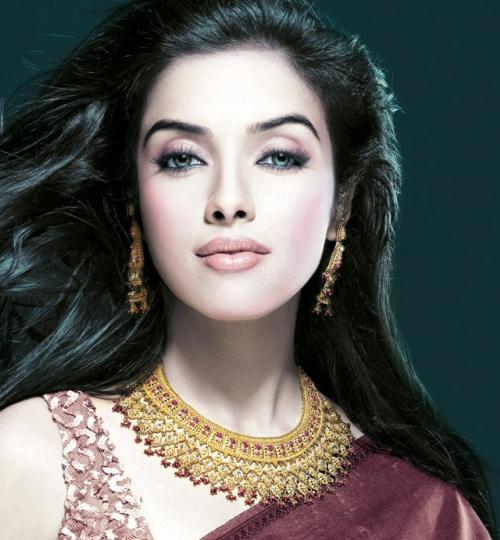
Dynamically replacing image map
To go further, we must dynamically assign a new image map when each new image replace another.
A simple example is a poster of the Battlestar Galactica series from which we want to view the photos of the two actresses.
The maps
A generic map serves as a container and is associated with the unique img tag.
<img id="img1" src="" width="" height="" border="0" usemap="#mapgeneric" >
<map name="mapgeneric" id="mapgeneric">
</map>
A map is created for the original image, the poster of the film:
<map name="mapgalactica" id="mapgalactica">
</map>
The contents of this map is copied into the generic map tag.
Another map is created for each new image.
The JavaScript code
A single JavaScript function replaces the images and the image map. This function is called when you click on an area defined by a map.
Example:
<area href="javascript:replaceImage('img1', 'url', 'name')" shape="rect" coords="0,0,170,350">
Function:
function replaceImage(imgid, source, mapid)
{
var image = document.getElementById(imgid);
image.src= source;
var newmap = document.getElementById(mapid);
var origin = document.getElementById("mapgeneric");
origin.innerHTML = newmap.innerHTML;
}
The function replaces the source of the image and use the innerHTML attribute to retrieve the contents of a map tag and assign it to the generic map associated with the img tag.
The first parameter is the ID of the img tag.
The second is the URL of the new image.
The third is the ID of the new image map.
- Interactive images with image map. How to navigate through successive images.