SetTimeout and setInterval: Delays in JavaScript
JavaScript can trigger action after an interval of time, or repeat it after an interval of time.
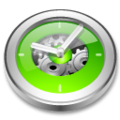
SetTimeout and setInterval methods are methods of the window object. We can then write setTimeout or window.setTimeout.
These are independent processes that, when they are initiated by an instruction, do not block the display of the rest of the page or the actions of the user.
SetTimeout indicates a delay before execution
The setTimeout method defines an action to perform and a delay before its execution. It returns an identifier for the process.
var x = setTimeout ("instructions" delay in milliseconds).
Example:
function myfonction() { ... }
setTimeout(myfonction, 5000);
The function will be executed after 5 seconds (5000 milliseconds).
The delay begins at the moment where setTimeout is executed by the JavaScript interpreter, so from the loading of the page if the instruction is in a script executed at load, or from a user action if the script is triggered by it.
ClearTimeout interrupts the count of setTimeout
This method stops the process started with the execution delay and the code associated with this delay.
The process to kill is recognized by the identifier returned by setTimeout.
Syntax:
clearTimeout(identifier);
Example:
var x = setTimeout(myfonction, 5000);
...
clearTimeout(x);
setInterval triggers an operation at regular intervals
Similar to setTimeout, it triggers the same action repeatedly at regular intervals.
setInterval ("instructions", delay)
Example:
setInterval ("alert ((beep)", 10000);
Displays a message every ten seconds.
The action will be repeated until you leave the page or clearInterval be executed.
clearInterval stops the action of setInterval
Stops the process triggered by setInterval.
Example:
var x = setInterval(myfunction, 10000);
...
clearInterval(x);
A lambda function may be a parameter
We can define a function inside the setTimeout or setInterval arguments.
Example:
var x = setTimeout(function() { alert("bip"); }, 2000);
The interest here is to use a recursive function, that can be done by referring to the function by the arguments.callee variable.
Demonstration
We will use setInterval to display the count of seconds.
The finish function after 10 seconds will display the message "THE END" and stop the countdown by calling clearInterval.
Click on the button to begin:
Source code:
<button onclick="start()"> Start the countdown </button>
<div id="bip"></div>
<script>
var counter = 10;
var intervalId = null;
function finish() {
clearInterval(intervalId);
document.getElementById("bip").innerHTML = "THE END!";
}
function bip() {
if(counter == 0) finish();
else {
document.getElementById("bip").innerHTML = counter + " seconds remaining";
}
counter--;
}
function start() { intervalId = setInterval(bip, 1000);
}
</script>
More demonstrations...
- setTimeout and setInterval.
How to modify the content of a page with a time interval or a given delay. - Triggering event at a given time.
How to simulate the Unix cron in JavaScript.